Making Frontend Testing Effective (or even Enjoyable)

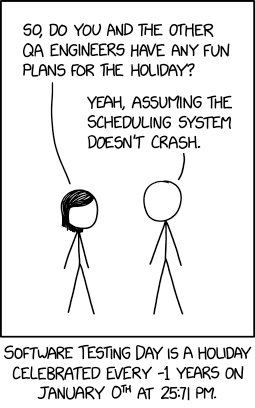
Let's be honest, the phrase "frontend testing" often evokes groans. For many developers, it conjures images of brittle, slow, and frustrating test suites that seem to break with every minor refactor. It's often seen as a necessary evil, a chore to be endured rather than a valuable part of the development process.
But what if it didn't have to be that way? What if frontend testing could actually be... dare I say... enjoyable? Or at the very least, significantly less painful and vastly more effective? The good news is, with the right mindset and modern tooling, it absolutely can be.
The core idea, heavily influenced by the philosophy behind tools like Testing Library, is simple yet powerful: Test your application the way your users interact with it.
From Implementation Details to User Experience
Historically, many frontend tests focused heavily on implementation details. We tested component internals, checked specific state values, or asserted on CSS class names. This approach leads to brittle tests. A simple code refactor, which doesn't change the user-facing behavior at all, could break dozens of tests, leading to wasted time and eroding confidence in the test suite.
The modern approach flips this script. Instead of asking "Is this component's state set correctly?", we ask "Can the user log in?", "Does clicking this button display the expected information?", "Is this error message visible when the form is submitted incorrectly?".
This user-centric approach means querying the DOM in ways similar to how a user (or assistive technology) would find elements – by visible text, labels, roles, etc. This makes tests more resilient to refactoring and provides genuine confidence that the application works as intended from the user's perspective.
Modern Testing Stack
Achieving this user-centric testing nirvana requires the right tools. The ecosystem has evolved significantly, offering solutions that prioritize developer experience (DX) and testing effectiveness:
-
Fast Test Runner (e.g., Vitest): Slow tests kill productivity and discourage developers from running them frequently. Modern runners like Vitest leverage advances like native ESM and smart caching to provide near-instant feedback. Its compatibility with the Jest API makes migration easier, and its focus on speed and DX makes writing and running tests much smoother.
Example (Vitest): A basic test structure looks familiar and clean.
// login-form.test.ts import { describe, it, expect } from 'vitest' import { render, screen } from '@testing-library/react' // Or your framework import LoginForm from './LoginForm' describe('LoginForm', () => { it('should render email and password fields', () => { render(<LoginForm />) // We'll use Testing Library queries here expect(screen.getByLabelText(/email/i)).toBeInTheDocument() expect(screen.getByLabelText(/password/i)).toBeInTheDocument() }) it('should render a submit button', () => { render(<LoginForm />) expect(screen.getByRole('button', { name: /log in/i })).toBeInTheDocument() }) })
-
User-Centric Assertions (Testing Library): As mentioned, Testing Library (available for React, Vue, Svelte, etc.) is foundational. It provides utilities to query the DOM based on accessibility and visibility, guiding you towards writing tests that resemble user interactions and avoid implementation details.
Example (Testing Library): Testing user interaction.
// login-form.test.ts (continued) import { describe, it, expect, vi } from 'vitest' import { render, screen, fireEvent } from '@testing-library/react' import userEvent from '@testing-library/user-event' // More realistic events import LoginForm from './LoginForm' it('should allow typing into fields and submitting', async () => { const user = userEvent.setup() // Setup user-event const handleSubmit = vi.fn() // Mock function using Vitest render(<LoginForm onSubmit={handleSubmit} />) const emailInput = screen.getByLabelText(/email/i) const passwordInput = screen.getByLabelText(/password/i) const submitButton = screen.getByRole('button', { name: /log in/i }) // Simulate user typing await user.type(emailInput, '[email protected]') await user.type(passwordInput, 'password123') // Simulate user clicking await user.click(submitButton) // Assert that our mock submit handler was called expect(handleSubmit).toHaveBeenCalledTimes(1) expect(handleSubmit).toHaveBeenCalledWith({ email: '[email protected]', password: 'password123' }) })
-
Realistic API Mocking (Mock Service Worker - MSW): Testing components that fetch data often involves complex mocking of fetch functions or libraries. MSW takes a different approach by intercepting requests at the network level. This means your component code (using
fetch
,axios
, etc.) runs exactly as it would in production. Your tests define mock responses for specific API endpoints.Example (MSW): Setting up a mock API handler for tests.
// src/mocks/handlers.js import { http, HttpResponse } from 'msw' export const handlers = [ // Intercept "POST /login" requests http.post('/login', async ({ request }) => { const info = await request.json() if (info.email === '[email protected]' && info.password === 'password123') { // Respond with a 200 status code and mock user data return HttpResponse.json({ userId: 'abc-123', name: 'Test User' }) } else { // Respond with an error status return new HttpResponse(null, { status: 401 }) } }), // Intercept "GET /user/abc-123" requests http.get('/user/abc-123', () => { return HttpResponse.json({ id: 'abc-123', name: 'Test User', email: '[email protected]' }) }) ] // In your test setup file (e.g., setupTests.ts) // import { setupServer } from 'msw/node' // import { handlers } from './mocks/handlers' // export const server = setupServer(...handlers) // beforeAll(() => server.listen()) // afterEach(() => server.resetHandlers()) // afterAll(() => server.close())
-
Reliable End-to-End Testing (e.g., Playwright): While component tests cover individual pieces, E2E tests verify complete user flows through the actual application running in a actual browser. Tools like Playwright offer speed, reliability, cross-browser testing, and powerful APIs for interacting with the page, making E2E testing less flaky and more valuable for critical paths.
Example (Playwright): Testing a login flow end-to-end.
// tests/login.spec.ts import { test, expect } from '@playwright/test' test('should allow a user to log in and see dashboard', async ({ page }) => { // Go to the login page await page.goto('/login') // Fill in the credentials await page.getByLabel(/email/i).fill('[email protected]') await page.getByLabel(/password/i).fill('password123') // Click the login button await page.getByRole('button', { name: /log in/i }).click() // Wait for navigation or expect an element on the dashboard page // Using URL change as an example await page.waitForURL('/dashboard') // Assert that the dashboard heading is visible await expect(page.getByRole('heading', { name: /dashboard/i })).toBeVisible() // Optional: Assert user-specific element is visible await expect(page.getByText(/welcome, test user/i)).toBeVisible() })
-
Visual Regression Testing: Sometimes, functional correctness isn't enough. UI bugs can creep in as subtle visual changes. Visual regression tools (like Playwright's built-in visual comparisons, Percy, Chromatic, etc.) take snapshots of your components or pages and compare them against baseline images, highlighting any unintended visual differences. This adds another layer of confidence, particularly for UI libraries or design systems. (Code examples for specific tools vary, but often involve a simple
expect(page).toHaveScreenshot()
or similar command).
Bringing It Together: Confidence and Velocity
By combining these elements – a user-centric philosophy, a fast runner like Vitest, user-focused assertions with Testing Library, realistic network mocking with MSW, and targeted E2E tests with Playwright – you create a testing strategy that delivers real value:
- Increased Confidence: Tests verify actual user behavior, giving you more confidence when shipping new features or refactoring.
- Improved Developer Experience: Fast feedback loops and tests that are easier to write and maintain reduce friction.
- Reduced Flakiness: Focusing on user-observable behavior and using robust tools minimizes brittle tests.
- Faster Development Cycles: Less time spent fixing broken tests or manually testing means faster iteration.
Frontend testing doesn't have to be a drag. By embracing modern tools and focusing on testing what truly matters – the user experience – we can build more robust applications, ship with greater confidence, and maybe, just maybe, find a little bit of joy in the process.